This guide provides an introduction to getting started with our APIs.
Starter mode
Pier’s Starter Mode allows you to use Pier for ledgering and compliance without Pier’s funds flow. Please see Starter Mode for more information.
Environments
The Pier API is accessible in two environments:
Environment | API url |
---|---|
Sandbox | sandbox.pier-finance.com/api |
Production | production.pier-finance.com/api |
The sandbox is designed to mimic the production environment, with a few exceptions. Loan agreements created in the sandbox will have large ‘draft’ watermarks and funds flows will only occur in production. Sandbox API keys are prefixed with test_
while production API keys are prefixed with prod_
. Otherwise, sandbox and production behavior is identical.
Postman Collection
You can fork our Postman Collection to immediately start interacting with the API. You’ll need to set a few variables to get started:
Variable | Description |
---|---|
clientId | Your API client ID (add this to the collection level Variables ) |
secret | Your API secret (add this to the collection level Variables ) |
token | The JWT token you get back from the /token endpoint (this goes in the Authorization header of each request; the Pre-request Script will set/update it for you) |
baseUrl | This should generally be set to https://sandbox.pier-finance.com/api |
When you open the collection click on the top level titled Pier Api
and choose Variables
. Create the variables clientId
/secret
and set the Current value
field (prevents credentials leaking). You are good to go! The Pre-request Script
will now automatically fill out the token
variable for each request:
This is a convenience that sets the token automatically each request and is simply calling the /token
endpoint like you would in your application.
OpenAPI Client Generation
Generation
Please see our OpenAPI Spec Repo to access our openapi.yml
spec file and generate a client in your language of choice. The following is an example on how to generate the typescript-axios
SDK using openapi-generator
on Mac:
Utilization
Here is an example of how to use the generated typescript-axios
SDK:
Authentication
Pier uses bearer auth to authenticate API requests. Call the /token
endpoint using client_id
and secret
in the post body to get a JWT token. The token should be included in the Authorization
header of all subsequent requests in the format of Bearer ${token}
.
Rate limits and retrying API requests
Only 5xx (Server Errors) and 429 (Rate Limits) errors should be retried.
Pier’s rate limit is set to 1,000 requests per minute. The limit applies to both the sandbox and production environments. If a rate limit is triggered, we will respond with a 429 status code.
Timezones
All loan-related items are in Pacific Time (PT). This includes things like first_payment_date
, next_payment_date
, all of the loan amortization schedules, etc. These times are usually date format (e.g. YYYY-MM-DD
) and simply determine the payment/accrual dates. All other (not payment related) timestamps are UTC and will follow the ISO8601
format.
Contributing
Feel free to contribute a pull request to our docs repo if you find anything that can be approved. We appreciate your help making our docs better!
Platform Status
See Pier’s Status Page for platform availability and feel free to subscribe for updates.
High-level origination flow
The following diagram outlines how the Pier origination flow works with the Borrower, Application, Loan Agreement and Facility resources:
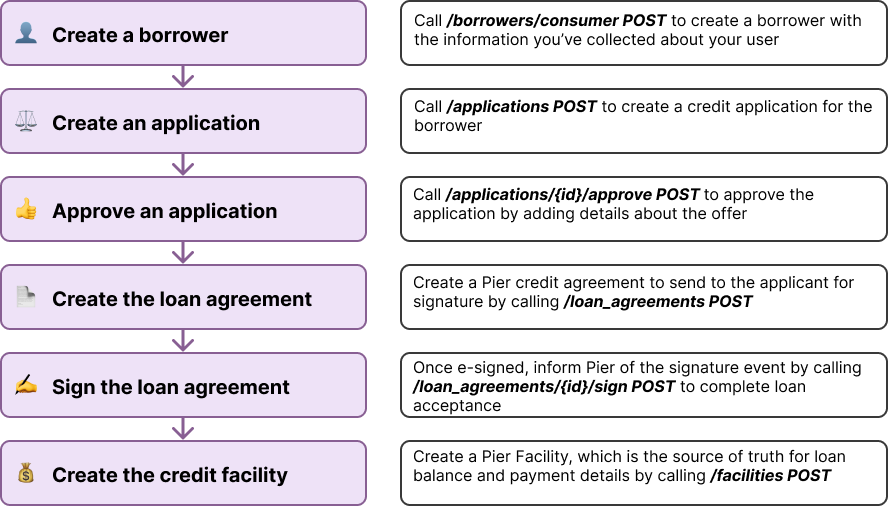